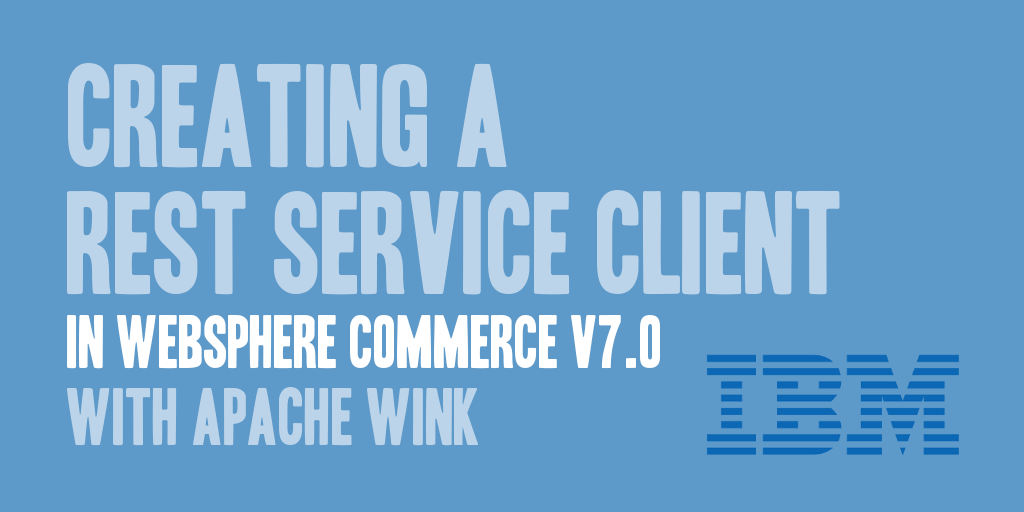
In WebSphere Commerce v7.0 inbound REST services are JAX-RS REST services and are built on top of Apache Wink. The WebSphere Commerce Information Center has plenty of documentation on how to create a inbound REST service; however, when trying to create an outbound REST service client, you are left to own research and devices as to an implementation strategy.
Knowing that WebSphere Commerce v7.0 includes the Apache Wink framework; we can leverage this to create a REST service client that is also based upon Apache Wink, and leverages a framework pattern rather than just pieces of code put together to execute HTTP calls and retrieve the responses.
NOTE: The Apache Wink version included in WebSphere Commerce v7.0 is 1.0-incubating.
The code below is an example of a GET request that requests and receives a JSON string response. The Apache Wink framework also supports POST and applicable other request types, but for the purposes of this example a GET request will be leveraged.
Apache Wink REST Client Creation Tutorial for WebSphere Commerce v7.0
Step 1. Create a ClientConfig object. This object will be used to configure and construct a RestClient.
By default, the client uses the java.net.HttpURLConnection for issuing requests and responses; but the Apache HttpClient can also be used. In my experience with WebSphere Commerce v7.0, I recommend using the ApacheHttpClientConfig object as shown below to construct your ClientConfig object.
Configure and set appropriate properties on the ClientConfig object, such as the readTimeout and connectTimeout properties.
NOTE: After a ClientConfig object is used to construct a RestClient object, the ClientConfig object can no longer be modified and will result in a ClientConfigException error.
// import org.apache.wink.client.ApacheHttpClientConfig; // import org.apache.wink.client.ClientConfig; ClientConfig clientConfig = new ApacheHttpClientConfig(); clientConfig.readTimeout(readTimeout); clientConfig.connectTimeout(connectionTimeout);
Step 2. Create a RestClient with the ClientConfig object created and configured previously.
// import org.apache.wink.client.RestClient; RestClient restClient = new RestClient(clientConfig);
Step 3. Create a Resource object with your REST service URI from the REST client object. Configure and set appropriate properties on the Resource object, and add any required headers to the request. Ensure you set the appropriate MediaType you expect to receive in response to your REST service call.
// import org.apache.wink.client.Resource; // import javax.ws.rs.core.MediaType; Resource restResource = restClient.resource("http://www.example.com/some/rest/service"); restResource.accept(MediaType.APPLICATION_JSON); restResource.header("HeaderField", "HeaderValue");
Step 4. Execute a GET or POST call, and process the response by using the status code, response headers, or the response message body as needed.
// import org.apache.wink.client.ClientResponse; ClientResponse clientResponse = restResource.get(); int statusCode = clientResponse.getStatusCode(); String responseEntity = clientRepsonse.getEntity(String.class);
NOTE: Instead of calling the response.getEntity(String.class) to retrieve a String response, you can use any other class that has a valid javax.ws.rs.ext.MessageBodyReader object.
And there you go, you’ve implemented an Apache Wink REST service client, and can now process the response as your application requires.
For further information about creating a Apache Wink REST service client, you’ll want to reference the IBM WebSphere Application Server v7.0 Information Center, and see the article Implementing clients using the Apache Wink REST client. This article is targeted towards WebSphere Application Server, and not WebSphere Commerce, so take that into account when attempting to follow applicable tutorials.
Nice article, good thing to know.
One question related to WCS Rest API: do you know if there’s some configuration in WCS 7 Rest API to allow cross origin requests to Rest, when firing request via Ajax? I’m trying to build demo mobile app client for WCS 7 Rest API, but I’m getting this kind of error (WCS is remote server):
XMLHttpRequest cannot load /wcs/resources/store/10151/categoryview/@top. Origin localhost:8081 is not allowed by Access-Control-Allow-Origin.
Pingback: Adding a Custom Entity Provider to an Apache Wink REST Service Client | @daharveyjr