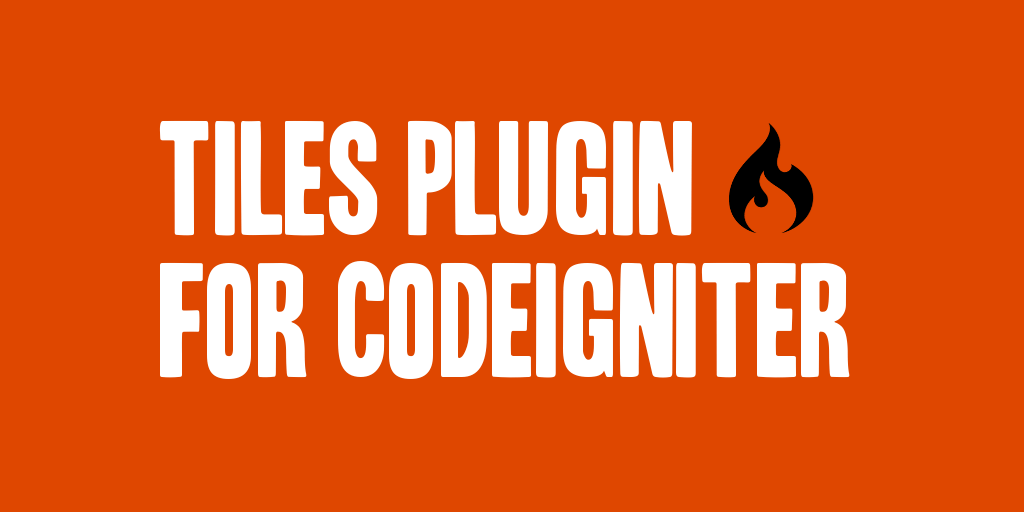
For anyone who has ever worked with Struts, it’s more than likely that you’ve used Tiles. Now granted you are not required to, but as most people can tell you it significantly eases development of your pages allowing you to focus on actual page content rather worry about your template code on each and every page.
With that in mind I have developed a tiles plugin, I guess you could say, for the CodeIgniter framework. I use it exclusively in my CodeIgniter projects as it helps me to put together pages quicker and more effectively. My goal of this post is to explain what I have done, it’s structure, setup and configuration, as well as provide the source for those who would like to use it within their own projects.
There are several pieces to this plugin. First you’ll extend the default Loader class included in CodeIgniter. Second, you’ll setup the folder and file structure necessary to support the tiles within your application. Third, you’ll need to setup your controller functions to call the tile method, rather than the traditional CodeIgniter view method.
First, I’ve started by extending the default Loader class to create a new function called tile that will be used to load our pages within a tiles template. I’ve done this to continue to allow the use of the view function, for ajax or other necessary requests, but ensured the tiles functionality can be used. You’ll find the code for the class below. Our tiles will leverage five (5) content areas that will be loaded for each tileset or template defined. These can obviously be changed, but for this release I’ve included the following content areas:
- Base: The presentation logic and code for the template base. All other content areas are populated into this template base.
- Header: The presentation logic and code for the template header area.
- Navigation: The presentation logic and code for the template navigation area.
- Footer: The presentation logic and code for the template footer area.
- Sidebar: The presentation logic and code for the template sidebar area.
- Content: The necessary view to be rendered as the actual content of the page. The presentation logic and code for the template content area.
The tile function will by default load the defined content areas, unless you override them as part of the data passed through to the function call. This allows for specific needs as required by your application, while maintaining a necessary base template. The function will also take any data passed through, as you would to a standard view, and ensure that data is passed through to all content views. In addition the function will pull any validation errors encountered by the form_validation library for display within the tiles template.
<!--?php if ( ! defined('BASEPATH')) exit('No direct script access allowed'); /** * MY_Loader Class * * @author Drew Harvey (http://www.daharveyjr.com/) * * This class extends the CI_Loader class, and adds tiles functionality. */ class MY_Loader extends CI_Loader { protected $_tilesets = array(); function __construct() { parent::__construct(); $this--->_tilesets = array( 'base' => array( 'base' => 'tiles/base/base', 'header' => 'tiles/base/header', 'navigation' => 'tiles/base/navigation', 'footer' => 'tiles/base/footer', 'sidebar' => 'tiles/base/sidebar' ) ); } /** * Load Tile * * This function is used to load a "tile" file. It has three parameters: * * 1. The name of the "tile" file to be included. * 2. An associative array of data to be extracted for use in the tile. * * @access public * @param string * @param array * @return void */ function tile($tileset = 'base', $view, $vars = array(), $return_flag = false) { $data = array(); // Get CI instance $CI =& get_instance(); $vars['error_string'] = validation_errors(); $vars['flash_message'] = $CI->session->flashdata('flash_message'); unset($CI); // Meta Checks $vars['meta_keywords'] = (!empty($vars['meta_keywords'])) ? $vars['meta_keywords'] : ''; $vars['meta_description'] = (!empty($vars['meta_description'])) ? $vars['meta_description'] : ''; // Create Response & Tile if (!isset($vars['title'])) { $data['title'] = "Ignite Reviews"; } else { $data['title'] = $vars['title']; } if (!isset($vars['navigation'])) { $vars['navigation'] = $this->view($this->_tilesets[$tileset]['navigation'], $vars, true); } else { $vars['navigation'] = $this->view($vars['navigation'], $vars, true); } if (!isset($vars['header'])) { $vars['header'] = $this->view($this->_tilesets[$tileset]['header'], $vars, true); } else { $data['header'] = $this->view($vars['header'], $vars, true); } if (!isset($vars['footer'])) { $data['footer'] = $this->view($this->_tilesets[$tileset]['footer'], $vars, true); } else { $data['footer'] = $this->view($vars['footer'], $vars, true); } if (!isset($vars['sidebar'])) { $vars['sidebar'] = $this->view($this->_tilesets[$tileset]['sidebar'], $vars, true); } else { $data['sidebar'] = $this->view($vars['sidebar'], $vars, true); } $data['content'] = $this->view($view, $vars, true); return $this->view($this->_tilesets[$tileset]['base'], $data, $return_flag); } } /* End of file MY_Loader.php */
Second, you’ll need to setup the appropriate folder and file structure to facilitate the tiles. Within your application/views folder, create a tiles folder. This folder will contain all template and content area files. For our ‘base’ template, we’ll specify a ‘base’ folder within the tiles folder, and then create the individual content area files within the ‘base’ folder. These content files you’ll customize to meet your specific UI and application needs.
Third, To call the tile function from your controller commands you’ll use it in the following context. Replace the ‘base’ variable with the name of the template you want to use. ‘view/to/display’, is the content view that you wish to populate into the template. It is identical to the view call, with one exception. You preface this call with the name of the tiles template that you wish to use.
$this->load->tile('base', 'view/to/display', $data);
You’ll notice that as part of the tiles plugin, I’ve allowed you to specify three particular pieces of data to pass through as part of your data array as well that will be picked up by the tiles plugin: meta_keywords, meta_description, and title. You can add more as your needs dictate, but I’ve found these three to be the most standard data for each application I’ve developed.
* To download the source code for this tiles plugin, please visit the CodeIgniter Tiles Plugin page, where you can find download links to the the plugin.
** Also note that for the example source code that is provided as part of this tiles plugin, you’ll need to enable the form_validation library and the session library. I’ve configured them to load automatically on every request through the autoload.php configuration file. If you’ve downloaded the example application, they’re already configured.